LaravelとAWS SESを使ってAPIメール送信を行う(Mailableクラス&SimpleEmailService)
- 公開日
- 更新日
- カテゴリ:Laravel
- タグ:PHP,Laravel,AWS,composer,artisan,Mail,Mailgun,AmazonSES,Mailable,API
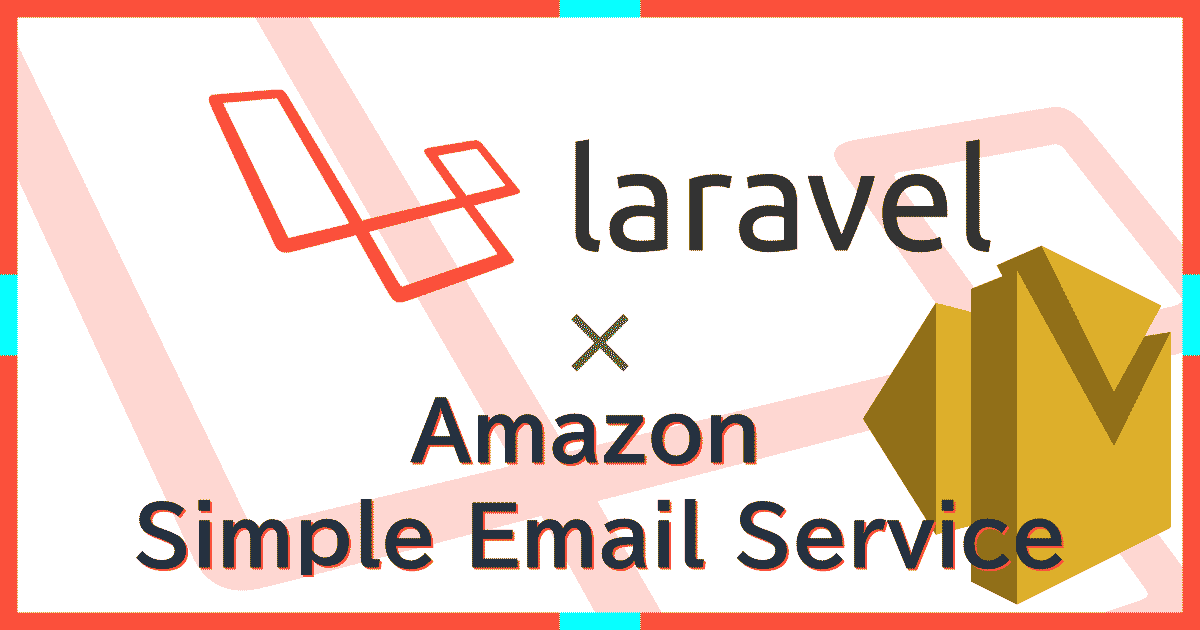
Laravel などの PHP フレームワークには、簡単にメール送信を行える機能が備わっています。
Laravel の場合はsmtp や sendmail はもちろんのこと、API ベースで送信を行うドライバも Amazon SES ・mailgun ・ mandrill ・ sparkpost と揃っています。
今回は、AWS の SES(SimpleEmailService) を使ったメール送信を行います。
Contents
開発環境
今回の環境については以下の通りです。
- Linux CentOS 7
- Apache 2.4
- PHP 7.1
- Laravel 5.5
Laravel のバージョンに関しては、5.5, 5.4, 5.3 であれば同一手順で進めていけます。
Laravel のルートディレクトリを「laravel/」としています。
また、Amazon SES でのメール送信を行いますので、最低限のセットアップは済んでいる前提で進めます。まだの場合は
AWS SES でのメール送信環境を構築する(Amazon Simple Email Service)
を参考にセットアップを行ってください。
そして、Amazon SES の API送信を扱える IAM ユーザを1つ用意しておいてください。
ライブラリ導入
まず初めに、Laravel で SES を使う為に必要な2つのライブラリを導入していきます。
Guzzle HTTP ライブラリ
Lravel で API ベースドライバを使う場合は、Guzzle HTTP ライブラリを導入する必要があります。
Laravel ルートディレクトリへ移動し、以下の composer コマンドを叩きます。
# Laravel ルートディレクトリへ移動する
cd /path/to/laravel
# Guzzle HTTP ライブラリを導入する
composer require guzzlehttp/guzzle
# 実行結果
[demo@localhost laravel]# composer require guzzlehttp/guzzle
Using version ^6.3 for guzzlehttp/guzzle
./composer.json has been updated
Loading composer repositories with package information
Updating dependencies (including require-dev)
Package operations: 4 installs, 0 updates, 0 removals
- Installing guzzlehttp/promises (v1.3.1): Downloading (100%)
- Installing psr/http-message (1.0.1): Downloading (100%)
- Installing guzzlehttp/psr7 (1.4.2): Downloading (100%)
- Installing guzzlehttp/guzzle (6.3.0): Downloading (100%)
Writing lock file
Generating optimized autoload files
> Illuminate\Foundation\ComposerScripts::postAutoloadDump
> @php artisan package:discover
Discovered Package: fideloper/proxy
Discovered Package: laravel/tinker
Package manifest generated successfully.
laravel/composer.json を開いて、require セクションを確認します。
"require": {
"php": ">=7.0.0",
"doctrine/dbal": "^2.6",
"fideloper/proxy": "~3.3",
"guzzlehttp/guzzle": "^6.3", // ← ココ
"laravel/framework": "5.5.*",
"laravel/tinker": "~1.0"
},
Guzzle HTTP ライブラリがインストールされました。
Amazon AWS SDK for PHP
Amazon SES ドライバを使用するには Amazon AWS SDK for PHP を導入する必要があります。こちらは、composer でインストールします。
laravel/composer.json の require セクションに以下を追記します。
"require": {
"php": ">=7.0.0",
"doctrine/dbal": "^2.6",
"fideloper/proxy": "~3.3",
"guzzlehttp/guzzle": "^6.3",
"laravel/framework": "5.5.*",
"laravel/tinker": "~1.0",
"aws/aws-sdk-php": "~3.0" // ← ココ
},
Laravel ルートディレクトリへ移動し、以下の composer コマンドを叩きます。
# Laravel ルートディレクトリへ移動する
cd /path/to/laravel
# composer コマンドで Amazon AWS SDK for PHP を導入する
composer update
# 実行結果
[demo@localhost laravel]# composer update
Loading composer repositories with package information
Updating dependencies (including require-dev)
Package operations: 2 installs, 0 updates, 0 removals
- Installing mtdowling/jmespath.php (2.4.0): Downloading (100%)
- Installing aws/aws-sdk-php (3.52.2): Downloading (100%)
aws/aws-sdk-php suggests installing aws/aws-php-sns-message-validator (To validate incoming SNS notifications)
Writing lock file
Generating optimized autoload files
> Illuminate\Foundation\ComposerScripts::postAutoloadDump
> @php artisan package:discover
Discovered Package: fideloper/proxy
Discovered Package: laravel/tinker
Package manifest generated successfully.
ドライバを SES へ切り替える
Laravel のメールドライバを SES へ切り替えていきます。
env ファイルに以下の設定項目を定義します。
- laravel/.env
-
MAIL_DRIVER=ses MAIL_FROM_ADDRESS=mail@code-tech.work MAIL_FROM_NAME=Laravel SES_KEY=YOURSESKEY SES_SECRET=YOURSESSECRET SES_REGION=us-east-1
services設定ファイルの SES部分を以下に変更します。
- config/services.php
-
'ses' => [ 'key' => env('SES_KEY'), 'secret' => env('SES_SECRET'), 'region' => env('SES_REGION'), ],
メールテンプレート作成
メールテンプレートを作成します。今回は HTML メールと平文メール両方を用意しますので、以下のような階層でディレクトリを作成し、それぞれ blade ファイルを作成します。
laravel
├─ resources
│ ├─ views
│ │ ├─ mail
│ │ │ ├─ html
│ │ │ │ └─ sesmail.blade.php
│ │ │ └─ plain
│ │ │ └─ sesmail.blade.php
laravel/resources/views 配下に mail/(html|plain)ディレクトリを作成し、それぞれ sesmail.bale.php を作成します。
作成した2つの Blade テンプレートファイルは、それぞれ以下のように記述します。
- laravel/resources/views/mail/html/sesmail.blade.php
-
<!DOCTYPE html> <html lang="ja"> <style> body { background-color: #2a88bd; color: #FFFFFF; } h1 { font-size: 16px; color: #ff6666; } </style> <body> <h1> Sample Notification from Amazon SES </h1> <p> Laravel & Amazon SES からメールが送信されました。 </p> <p> <a href="https://www.ritolab.com">https://www.ritolab.com</a> </p> </body> </html>
- laravel/resources/views/mail/plain/sesmail.bale.php
-
Sample Notification from Amazon SES Laravel & Amazon SES からメールが送信されました。 https://www.ritolab.com
mailable クラス作成
Laravel でのメール送信クラスである mailable クラスを作成していきます。
mailable クラス生成
まずは mailable クラスを生成します。 Laravel ルートディレクトリへ移動し、以下の artisan コマンドを叩きます。
# Laravel ルートディレクトリへ移動する
cd /path/to/laravel
# artisan コマンドで mailable クラスを生成する
php artisan make:mail SampleSesMailable
# 実行結果
[demo@localhost laravel]# php artisan make:mail SampleSesMailable
Mail created successfully.
laravel/app/Mail 配下に SampleSesMailable.php が生成されます。
laravel
├ app
│ ├─ Mail
│ │ └─ SampleSesMailable.php
- laravel/app/Mail/SampleSesMailable.php
-
<?php namespace App\Mail; use Illuminate\Bus\Queueable; use Illuminate\Mail\Mailable; use Illuminate\Queue\SerializesModels; use Illuminate\Contracts\Queue\ShouldQueue; class SampleSesMailable extends Mailable { use Queueable, SerializesModels; /** * Create a new message instance. * * @return void */ public function __construct() { // } /** * Build the message. * * @return $this */ public function build() { return $this->view('view.name'); } }
mailable クラス実装
それでは生成した mailable クラスを実装していきます。以下のようになります。
- laravel/app/Mail/SampleSesMailable.php
-
<?php namespace App\Mail; use Illuminate\Bus\Queueable; use Illuminate\Mail\Mailable; use Illuminate\Queue\SerializesModels; use Illuminate\Contracts\Queue\ShouldQueue; class SampleSesMailable extends Mailable { use Queueable, SerializesModels; protected $title; /** * Create a new message instance. * * @return void */ public function __construct() { $this->title = 'Amazon SESからのメール送信テストです。'; } /** * Build the message. * * @return $this */ public function build() { return $this->view('mail.html.sesmail') ->text('mail.plain.sesmail') ->subject($this->title); } }
メール画面プレビュー
送信を行う前に、先ほど作ったテンプレートから、メール画面をプレビューしてみます。ルーティングを以下のように定義します。
- laravel/routes/web.php
-
Route::get('sample/ses/preview', function () { return new App\Mail\SampleSesMailable(); });
記述できたら、ブラウザから
http://YOUR-DOMAIN/sample/ses/preview
へアクセスしてみます。
HTML メールではこんな感じで見えることになります。
動作確認
それでは実際にメールを送信してみます。
ルーティングに以下を追加します。
use Illuminate\Support\Facades\Mail;
use App\Mail\SampleSesMailable;
Route::get('sample/ses/send', function () {
$to = 'test@gmail.com';
Mail::to($to)->send(new SampleSesMailable());
});
記述できたら、ブラウザから
http://YOUR-DOMAIN/sample/ses/send
へアクセスします。
アクセスしたらメールを確認してみます。
問題なくメールが送信されました。
また、平文メールもこんな感じです。
まとめ
以上で作業は完了となります。
Amazon SES は API メールの中でも特に優秀だと思います。セットアップも簡単で、無料送信分も個人で使うには十分です。特に AWS で WEB アプリケーションを運用している場合には Laravel と SES の組み合わせはとてもおすすめです。
なにより、いちいち SMTP サーバを構築しなくても良いのがいいですね。最近は受信も行えるようになった Amazon SES にはこれからも注目です。是非試してみてください。